Home »
Aptitude Questions and Answers »
C Aptitude Questions and Answers
C Structure and Union - Aptitude Questions & Answers
C programming Structure and Union Aptitude Questions and Answers: In this section you will find C Aptitude Questions and Answers on Structure and Union Questions.
List of C programming Structure and Union Aptitude Questions and Answers
1) What will be the output of following program ?
#include <stdio.h>
struct sample
{
int a=0;
char b='A';
float c=10.5;
};
int main()
{
struct sample s;
printf("%d,%c,%f",s.a,s.b,s.c);
return 0;
}
- Error
- 0,A,10.5
- 0,A,10.500000
- No Error , No Output
Correct Answer - 1
Error: Can not initialize members here
We can only declare members inside the structure, initialization of member with declaration is not allowed
in structure declaration.
2) What will be the output of following program ?
#include <stdio.h>
int main()
{
struct sample{
int a;
int b;
sample *s;
}t;
printf("%d,%d",sizeof(sample),sizeof(t.s));
return 0;
}
- 12,12
- 12,0
- Error
- 12,4
Correct Answer - 4
12,4
There are 3 members with in structure (a,b,s), a and b is an integer variable that takes
4-4 bytes in memory, s is self-refrencial pointer of sample and pointer also takes 4 bytes in memory.
3) What will be the output of following program ?
#include <stdio.h>
#include < string.h >
struct student
{
char name[20];
}std;
char * fun(struct student *tempStd)
{
strcpy(tempStd->name,"Thomas");
return tempStd->name;
}
int main()
{
strcpy(std.name,"Mike ");
printf("%s%s",std.name,fun(&std));
return 0;
}
- Mike Thomas
- Mike Mike
- ThomasThomas
- ThomasMike
Correct Answer - 3
ThomasThomas
4) What will be the output of following program ?
#include <stdio.h>
struct sample
{
int a;
}sample;
int main()
{
sample.a=100;
printf("%d",sample.a);
return 0;
}
- 0
- 100
- ERROR
- Warning
Correct Answer - 2
100
This type of declaration is allowed in c.
5) What will be the output of following program ?
#include <stdio.h>
struct employee{
int empId;
char *name;
int age;
};
int main()
{
struct employee emp []={ {1,"Mike",24}, {2,"AAA",24}, {3,"BBB",25}, {4,"CCC",30} };
printf("Id : %d, Age : %d, Name : %s", emp[2].empId,3[emp].age,(*(emp+1)).name);
return 0;
}
- Id : 3, Age : 24, Name : Mike
- Id : 3, Age : 23, Name : Mike
- Id : 3, Age : 30, Name : AAA
- ERROR
Correct Answer - 3
Id : 3, Age : 30, Name : AAA
6) What will be the output of following program ? (On 16 bit compiler)
#include <stdio.h>
int main()
{
union values
{
int intVal;
char chrVal[2];i
};
union values val;
val.chrVal[0]='A'; val.chrVal[1]='B';
printf("\n%c,%c,%d",val.chrVal[0],val.chrVal[1],val.intVal);
return 0;
}
- A,B,0
- A,B,16961
- B,B,66
- A,A,65
Correct Answer - 2
A,B,16961
Only 2 bytes will be occupied for this union variable val.
Data will store in memory like this :
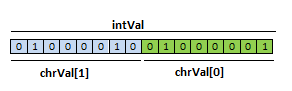
So the value of intVal is
01000010 01000001 is equivalent to
16961.
7) What will be the output of following program ? (On 16 bit compiler)
#include <stdio.h>
int main()
{
union values
{
unsigned char a;
unsigned char b;
unsigned int c;
};
union values val;
val.a=1;
val.b=2;
val.c=300;
printf("%d,%d,%d",val.a,val.b,val.c);
return 0;
}
- 44,44,300
- 1,2,300
- 2,2,300
- 256,256,300
Correct Answer - 1
44,44,300
Only 2 bytes will be occupied for this union variable val.
Data will store in memory like this :
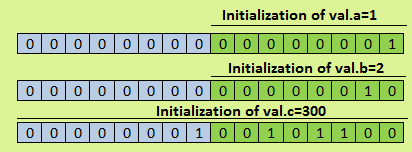
in printf() statement val.a and val.b will print the value of first Byte ( 8 bits) because,
a and b are character and it takes one Byte only, but val.c will orint the value of 2 bytes.
So, value of val.a and val.b will be
00101100 (44) and value of val.c will be
00000001 00101100 (300).
8) What will be the output of following program ? (On 16 bit compiler)
#include <stdio.h>
int main()
{
typedef struct tag{
char str[10];
int a;
}har;
har h1,h2={"IHelp",10};
h1=h2;
h1.str[1]='h';
printf("%s,%d",h1.str,h1.a);
return 0;
}
- ERROR
- IHelp,10
- IHelp,0
- Ihelp,10
Correct Answer - 2
Ihelp,10
It is possible to copy one structure variable into another like h1=h2. Hence value of h2.str
is assigned to h1.str.
9) What will be the output of following program ?
#include <stdio.h>
int main()
{
struct std
{
char name[30];
int age;
};
struct std s1={"Mike",26};
struct std s2=s1;
printf("Name: %s, Age: %d\n",s2.name,s2.age);
}
- Name: Mike, Age: 26
- Name: Garbage, Age: Garbage
- Error
Correct Answer - 1
Name: Mike, Age: 26
A structure variable can be assigned like this struct std s2=s1; if structure variables are same.
10) What will be the output of following program ?
#include <stdio.h>
int main()
{
union test
{
int i;
int j;
};
union test var=10;
printf("%d,%d\n",var.i,var.j);
}
- 10,10
- 10,0
- 0,10
- Error
Correct Answer - 4
Error: Invalid Initialization
You cannot initialize an union variable like this.